How To Integrate Relay Protocol API in Your dApp (with Code Examples)
Learn how to integrate Relay Protocol’s API into your dApp with full code examples. Enable seamless token swaps and cross-chain bridging between Ethereum, Optimism, and 70+ blockchains. Developer-friendly, scalable, and easy to implement.
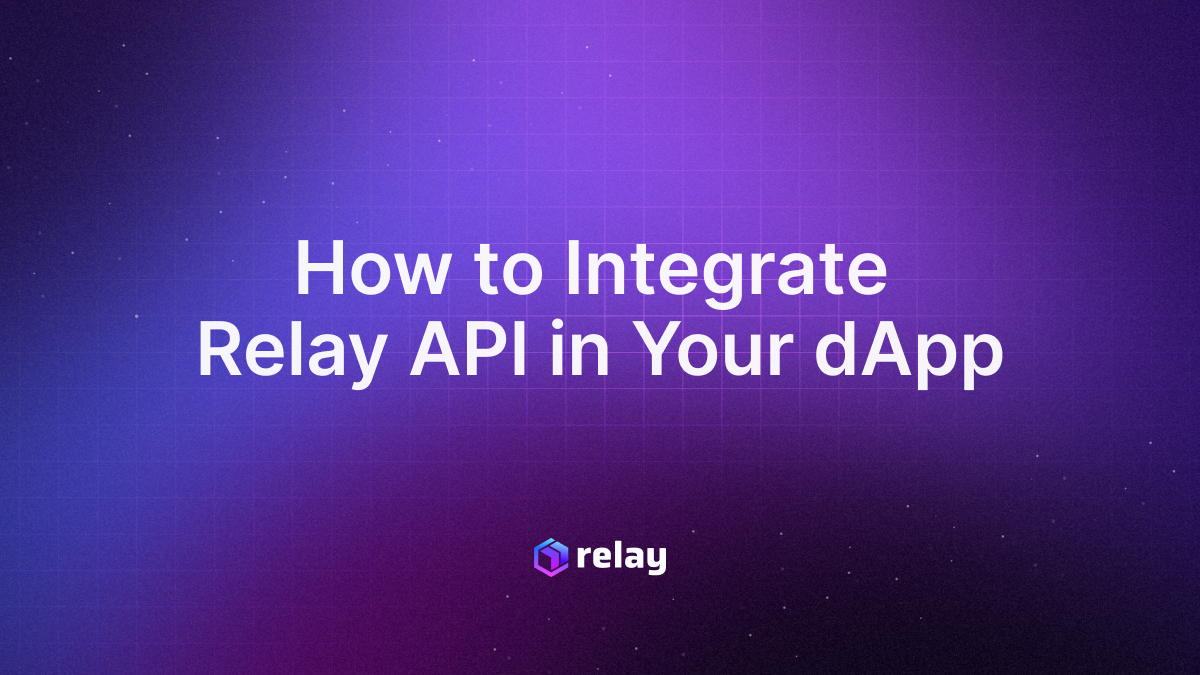
Seamless cross-chain functionality is crucial for increasing adoption in decentralized applications (dApps). The Relay API offers a powerful, developer-friendly solution for integrating token swaps and bridging assets across major blockchains like Ethereum and Optimism.
In this guide, you'll learn how to integrate Relay’s API into your dApp with step-by-step instructions and working code examples—empowering you to create a user-friendly cross-chain experience.
Why Build on Relay Protocol?
Relay Protocol provides a high-performance, low-friction layer for cross-chain bridging and swaps across 72+ blockchains, featuring:
- Cross-Chain Simplicity: Swap ETH to USDC across chains with minimal code.
- Developer-Friendly APIs: Clear endpoints like
POST /quote
and solid SDK support. - High Scalability: Ideal for DeFi, gaming, and high-volume apps.
Get started by requesting an API key at relay.link.
Prerequisites
Before diving into the integration, make sure you have:
- Node.js (v16+)
- A test wallet like MetaMask
- Relay API Key (from relay.link)
- Basic JavaScript/TypeScript knowledge
- Axios and Viem libraries installed
Step 1: Set Up Your dApp Project
Start by creating your project environment.
mkdir relay-api-dapp
cd relay-api-dapp
npm init -y
npm install axios viem dotenv
Add a .env
file with your Relay API key:
RELAY_API_KEY=your_relay_api_key
Test the environment setup:
// index.js
require("dotenv").config();
console.log("Relay API Key Loaded:", !!process.env.RELAY_API_KEY);
Step 2: Authenticate with Relay API
Set up an Axios instance to communicate with the Relay API:
// utils/relayApi.js
const axios = require("axios");
const relayApi = axios.create({
baseURL: process.env.RELAY_API_KEY.includes("testnet")
? "https://api-testnet.relay.link"
: "https://api.relay.link",
headers: {
Authorization: `Bearer ${process.env.RELAY_API_KEY}`,
"Content-Type": "application/json",
"x-relay-source": "my-dapp",
},
});
module.exports = relayApi;
Test the connection:
// index.js
const relayApi = require("./utils/relayApi");
async function testRelayApi() {
try {
const response = await relayApi.get("/chains");
console.log("Relay Protocol Chains:", response.data);
} catch (error) {
console.error("Relay API Error:", error.response?.data || error.message);
}
}
testRelayApi();
Step 3: Fetch Cross-Chain Quotes
Use Relay's POST /quote
API to get transaction plans for token swaps.
// utils/relayApi.js
async function getQuote(params) {
try {
const response = await relayApi.post("/quote", params);
if (!response.data.steps) throw new Error("No swap route available");
return response.data;
} catch (error) {
throw new Error(`Get Quote Error: ${error.response?.data?.message || error.message}`);
}
}
module.exports = { relayApi, getQuote };
Example usage:
// index.js
const { getQuote } = require("./utils/relayApi");
async function fetchCrossChainQuote() {
const params = {
fromChainId: 1,
toChainId: 10,
fromToken: "0x0000000000000000000000000000000000000000",
toToken: "0x7f5c764cbc14f9669b88837ca1490cca17c31607",
amount: "10000000000000000",
wallet: "0xYourTestWalletAddress",
};
try {
const quote = await getQuote(params);
console.log("Cross-Chain Quote:", quote);
} catch (error) {
console.error("Quote Error:", error.message);
}
}
fetchCrossChainQuote();
Step 4: Execute Transactions with Step Execution API
Create a wallet client using Viem:
// utils/wallet.js
const { WalletClient, http } = require("viem");
const { privateKeyToAccount } = require("viem/accounts");
const { mainnet } = require("viem/chains");
function createWalletClient(privateKey) {
const account = privateKeyToAccount(privateKey);
return WalletClient.create({
account,
chain: mainnet,
transport: http(),
});
}
module.exports = { createWalletClient };
Now execute the swap steps:
// utils/relayApi.js
async function executeSteps(quote, walletClient) {
for (const step of quote.steps) {
if (step.kind === "transaction") {
try {
const txHash = await walletClient.sendTransaction({
to: step.to,
data: step.data,
value: BigInt(step.value || 0),
});
const receipt = await walletClient.waitForTransactionReceipt({ hash: txHash });
if (receipt.status === "reverted") throw new Error(`Transaction ${txHash} reverted`);
console.log(`Step Transaction ${txHash} Successful`);
} catch (error) {
throw new Error(`Step Execution Failed: ${error.message}`);
}
}
}
return "Cross-Chain Swap Completed";
}
module.exports = { relayApi, getQuote, executeSteps };
Step 5: Implement Error Handling & Reliability
Robust dApps need strong validation and retry logic.
Input validation:
if (!process.env.TEST_PRIVATE_KEY) throw new Error("Missing TEST_PRIVATE_KEY");
if (!Number(params.amount) || Number(params.amount) <= 0) {
throw new Error("Invalid swap amount");
}
Retry failed steps:
async function executeSteps(quote, walletClient, retries = 2) {
for (const step of quote.steps) {
if (step.kind === "transaction") {
for (let attempt = 1; attempt <= retries; attempt++) {
try {
const txHash = await walletClient.sendTransaction({
to: step.to,
data: step.data,
value: BigInt(step.value || 0),
});
const receipt = await walletClient.waitForTransactionReceipt({ hash: txHash });
if (receipt.status === "reverted") throw new Error(`Transaction ${txHash} reverted`);
console.log(`Step Transaction ${txHash} Successful`);
break;
} catch (error) {
if (attempt === retries) {
throw new Error(`Step Execution Failed after ${retries} Attempts: ${error.message}`);
}
console.log(`Retrying Step (${attempt}/${retries})...`);
await new Promise((resolve) => setTimeout(resolve, 1000));
}
}
}
}
}
Step 6: Test Integration on Relay Testnet
Switch baseURL
to the testnet version:
baseURL: "https://api-testnet.relay.link",
Update parameters to testnet chains (e.g., Sepolia) and tokens. Fund your test wallet using a testnet faucet and run the test.
Step 7: Use Advanced Relay API Features
List supported tokens:
async function getCurrencies(chainId) {
const response = await relayApi.get(`/currencies?chainId=${chainId}`);
return response.data;
}
Track transaction status:
async function getExecutionStatus(requestId) {
const response = await relayApi.get(`/execution/status?requestId=${requestId}`);
return response.data;
}
Cross-chain interoperability is no longer a luxury—it’s a necessity. Relay Protocol empowers your dApp to deliver seamless token swaps and bridges between chains like Ethereum and Optimism using a simple yet powerful API suite.